Constrained double integrator¶
This example is intended to present PyTrajectory’s capabilities on handling system constraints. To do so, consider the double integrator which models the dynamics of a simple mass in an one-dimensional space, where a force effects the acceleration. The state space representation is given by the following dynamical system.
A possibly wanted trajectory is the translation from to
within
. At the beginning and end the mass should stay at rest, that is
.
Now, suppose we want the velocity to be bounded by .
To achieve this PyTrajectory needs a dictionary containing the index of the constrained variable in
and a tuple with the corresponding constraints. So, normally this would look like
>>> con = {1 : [0.0, 0.65]}
But, due to how the approach for handling system constraints is implemented, this would throw an exception because
the lower bound of the constraints is equal to
and has to be smaller.
So instead we use the dictionary
>>> con = {1 : [-0.1, 0.65]}
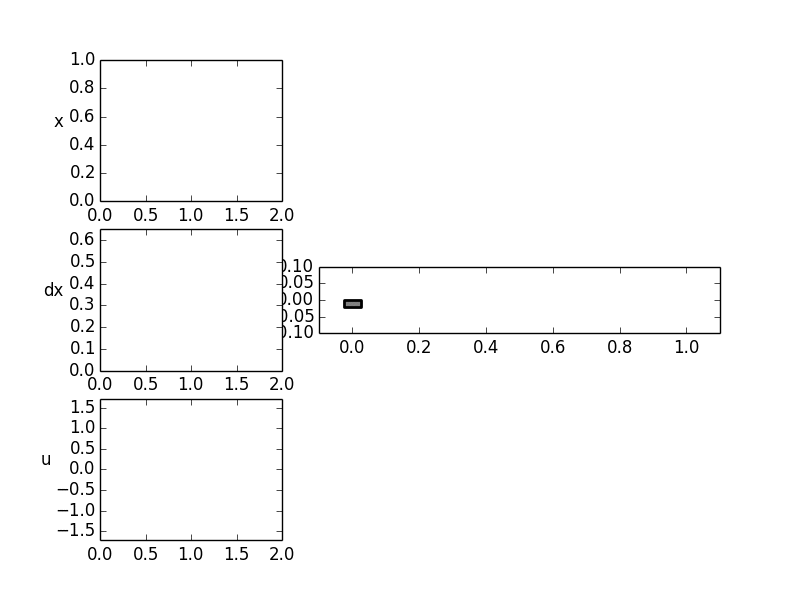
Source Code¶
'''
This example of the double integrator demonstrates how to pass constraints to PyTrajectory.
'''
# imports
from pytrajectory import ControlSystem
import numpy as np
# define the vectorfield
def f(x,u):
x1, x2 = x
u1, = u
ff = [x2,
u1]
return ff
# system state boundary values for a = 0.0 [s] and b = 2.0 [s]
xa = [0.0, 0.0]
xb = [1.0, 0.0]
# constraints dictionary
con = {1 : [-0.1, 0.65]}
# create the trajectory object
S = ControlSystem(f, a=0.0, b=2.0, xa=xa, xb=xb, constraints=con, use_chains=False)
# start
x, u = S.solve()